- Published on
Singleton
- Authors
- Name
- Arnaud Ferrand
Definition
- Ensure a class has only one instance, and provide a global point of access to it. [GoF, p127]
- Encapsulated "just-in-time initialization" or "initialization on first use".
UML class diagram
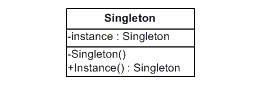
Participants
The classes and/or objects participating in this pattern are :
- Singleton defines an Instance operation that lets clients access its unique instance. Instance is a class operation. responsible for creating and maintaining its own unique instance.
Sample code
This code demonstrates the Singleton pattern which assures only a single instance (the singleton) of the class can be created.
Java:
public class Singleton {
private static Singleton _instance= null;
/**
* La présence d'un constructeur privé supprime
* le constructeur public par défaut.
*/
private Singleton() {}
/**
* Le mot-clé synchronized sur la méthode de création
* empêche toute instanciation multiple même par
* différents threads.
* Retourne l'instance du singleton.
*/
public synchronized static Singleton getInstance() {
if (_instance== null)
_instance= new Singleton();
return _instance;
}
}
C#:
// --------------------------------------------------------
//
// Creational Patterns : Singleton
//
// --------------------------------------------------------
using System;
namespace DesignPatterns
{
/// <summary>
/// The singleton class.
/// A single instance of the class can be created
/// </summary>
public class Singleton
{
/// <summary>
///
/// </summary>
private static Singleton instance = null;
/// <summary>
/// Private Constructor
/// </summary>
private Singleton()
{
}
/// <summary>
/// gets the singleton instance
/// </summary>
/// <returns>The singleton instance</returns>
public static Singleton Instance()
{
// 'Lazy initialization'
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
} // end class
/// <summary>
/// Testing class
/// </summary>
public class SingletonTest
{
public static void Main()
{
// Getting references to the Singleton
Singleton s1 = Singleton.Instance();
Singleton s2 = Singleton.Instance();
if (s1 == s2)
{
Console.WriteLine("References are the same. The objects share the same instance");
}
// Wait for user
Console.Read();
}
} // end class
}